If you have tried to run a PowerShell script before with SCCM you might have found it odd and not exactly intuitive. Here are a couple tips.
The most frustrating part of this problem is simply...not being able to tell what is wrong. The error message comes and goes before you have a chance of seeing it.
It isn't neccessary to do this (as I've already done it), but to solve the problem I modified my SCCM command line as follows:
%COMSPEC% /K powershell.exe -noprofile -file script.ps1 -executionpolicy Bypass
When running this the first thing you will notice is an error from cmd.exe saying UNC paths are not supported, reverting back to the windows directory. Well now your script wont launch because there is no correct working directory (which WOULD have been the network share on your distribution point). To get around THIS you have to set the following:
Key: HKLM\Software\Wow6432Node\Microsoft\Command Processor
Value: DisableUNCCheck
Data (DWORD): 1
Now if you run your program again you will see it says the execution of scripts on the local machine has been disabled. Luckily you have a hand dandy command prompt (thanks to the /K switch) so you can type powershell -command "Get-ExecutionPolicy -list".
You will see that everything is Undefined. If you go open up a regular command prompt and type the same thing, you should see whatever your actual settings are (in my case it was Bypass set to the LocalMachine scope and everything else undefined, this was set to Bypass for TESTING reasons).
A whoami in the SCCM kicked command prompt shows nt authority\system as you would expect.
So the problem appears to be that when run as the system account -executionpolicy is ignored and it doesn't appear to be getting/setting it's execution policy in the same place everything else is.
For instance, right now on the same machine I have two windows open, one powershell run as administrator (via a domain account in the local admins group), the other via the command prompt SCCM launches. Here are the Get-ExecutionPolicy -list results from each:
Local Admin:

SCCM
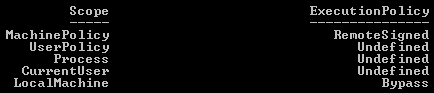
Same machine, two different settings. First attempt was to use:
powershell.exe -noprofile -command "Set-ExecutionPolicy Bypass LocalMachine" -File script.ps1
This failed and ultimately it appears that powershell will either run -command or -file, but not both.
So the solution to running PowerShell scripts as admin via SCCM is to do the following:
Create an SCCM Program with the following command line:
powershell.exe -noprofile -command "Set-ExecutionPolicy Bypass LocalMachine"
Then one with the following:
powershell.exe -noprofile -file script.ps1
And finally a cleanup program:
powershell.exe -noprofile -command "Set-ExecutionPolicy RemoteSigned LocalMachine"
Obviously RemoteSigned should be whatever your organization has decided as the standard Execution Policy level, the default I believe is Restricted, most will probably use RemoteSigned, security (over)conscious will probably use AllSigned.
Is this ideal? No. Of course not. It paying attention to the -ExecutionPolicy switch would be ideal.
But it works.
And on a bit of a side tangent, I think it is a pretty "microsoft" view of security to put this rather convoluted security system in place, and then still have VBScripts executable right out the gate on Windows 7 box. And batch files.
What is the point of all this obnoxious security when, at the end of the day...they can just use VBScript. Had they made you toggle a setting somewhere to enable VBScript and Batch files, had they not made 5 scopes of security policy for powershell, had they basically not admitted that they don't know how to do a secure scripting language (your security should probably come from the user rights, and not purely the execution engine, though, that's a finer point you could argue several ways), I'd probably be a lot more sympathetic.
Had SCCM not been such a myopic monolithic dinosaur, this wouldn't be a problem. These are all symptoms of what will eventually kill them. Legacy. 64-bit filesystem redirection, the registry as a whole, more specifically Wow6432Node, Program Files (x86), needing to use PowerShell, VBScript or Batch files to do a simple file transfer/shortcut placement.
This is System Center Configuration Manager. It can use bits to copy a multi-gigabyte install "safely" to a client machine...but only if it's then going to run an installer.
It can't copy a file and place a shortcut, or add a key to the registry, it only manages the configuration in the most outmoded and obsolete ways possible.
/rant
Update on 2012-03-08 23:05 by Paul Fulbright
Few quick notes. You see how MachinePolicy is set? That WILL override your SCCM packages, that means GPO is controlling the ExecutionPolicy, so while your command will take effect, it will be overruled.
Second note is the reason the two are different is because the SCCM version is using the x86 settings, not x64. Which, while it explains the difference, does not explain why running it with -ExecutionPolicy Bypass is ignored, nor why running a script as a user in SCCM works fine, but as an admin, it does not. End result being, you still need the workaround.